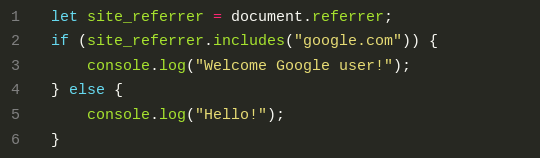
How to Get the Referrer in JavaScript
Whether it’s for analytics purposes or for any other reason, you sometimes need to know where a visitor to your site comes from.
In JavaScript, you can tell whether a string contains another string by using the includes()
function.
includes()
FunctionThe includes()
function requires a string and substring. If required, you can also pass an optional start position (more on this later).
The function returns true
if the string contains the substring, and false
otherwise.
|
|
To call the includes()
function, pass the following parameters:
my_string
is the string you would like to test.substring
is the substring you are looking for.start_position
if you would like to start searching at a specific location. If the substring is not found beyond that location,Most of the time, you will not need to have a start position. In that case, the function ends up being simpler:
|
|
includes()
FunctionWhile the includes()
function in JavaScript is fairly simple, it does come with a couple of quirks.
I mentioned an optional position
parameter a moment ago. Using the position
parameter is as simple as passing the function a positive integer.
|
|
That’s it. If you do this, the includes()
function will ignore the first letter, as its position is zero. It will search from that point on.
For example, let’s take a string such as "Hello world!"
. The letter H is in position 0, calling "Hello world!".includes("H", 1);
will return false. You can do this with just about any other letter. Be careful when counting, though, as mis-counting can yield unwanted results!
|
|
includes()
Function is Case Sensitive!As the title says, calling includes("FancyDev.net")
is not identical to calling includes("fancydev.net")
. Don’t believe it? Give the following code a try:
|
|
includes()
FunctionYou don’t need the includes()
function to be case sensitive. In fact, writing your own is rather easy.
You’ll need to do the following:
includes()
function with the new strings.That’s it! The following function is a case insensitive version of the includes()
function.
|
|
If in doubt, give it a try. This function will behave just like the standard includes()
function, but it will ignore the strings' capitalization.
Ignoring capitalization when testing strings can be useful, for example, when you’re coding an authentication or profile system. Most of time, you don’t want to have a user called my_user
and another user called MY_USER
on your site. That would only cause confusion.
Similarly, users sometimes capitalize their usernames and e-mail addresses when logging in; sometimes they do not. You don’t want to lock them out of their accounts based on how they capitalized their names. Having to unnecessarily re-type your password makes for an ugly user experience!
You can now use the includes()
function in a multitude of tasks. The above explanations, combined with the following example, should be enough to get you started. 😎
|
|
Whether it’s for analytics purposes or for any other reason, you sometimes need to know where a visitor to your site comes from.
If, for some reason, you need to write a Hugo shortcode on your website, you need to escape or inactivate it.